Interface Segregation Principle
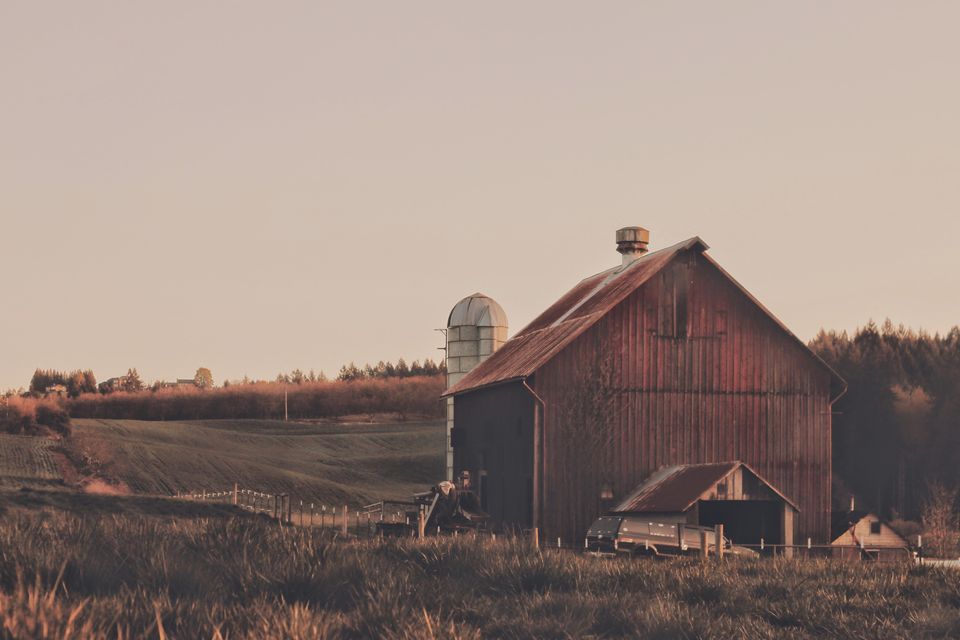
In the previous article we learned about the Liskov Substitution Principle. In this Article we will be covering the Interface Segregation Principle.
The Interface Segregation Principle, is the fourth SOLID Principle for OOP.
For those new to SOLID, S.O.L.I.D Stands for:
- Single Responsibility Principle
- Open Closed Principle
- Liskov Substitution Principle
- Interface Segregation Principle
- Dependency Inversion Principle
This article is the fourth of a five post series that will cover the SOLID Principles.
You shouldn’t force a Class to implement a method it has no use for
This is probably the simplest Principle to understand. It simply states, you shouldn't force a class to implement a method it has no use for. In other words, when creating your interfaces, make sure the interfaces are like classes, and have a single responsibility.
For example let's say we have the following interface.
Interface PlantInterface() {
Public function sum();
Public function getVitaminK();
}
Then when we create our plant classes we implement our PlantInterface.
Class Carrots Implements PlantInterface
{
public function sum()
{
...
}
public function getVitaminK()
{
...
}
}
And another plant:
Class Jicama Implements PlantInterface
{
public function sum()
{
...
}
public function getVitaminK()
{
...
}
}
Ok so far so good. Except we have a small technical problem. Jicama doesn't actually have Vitamin K. But since we have it included as a method in the Plant Interfaces, all plants have to declare the method, even if it's just an empty method. Well that doesn't make much sense does it?
The way to fix this is to make a separate interface.
Interface VitaminKInterface
{
public function getVitaminK(){}
}
Now our two plant Classes can look like:
Class Carrots Implements PlantInterface, VitaminKInterface
{
public function sum()
{
...
}
public function getVitaminK()
{
...
}
}
And another plant:
Class Jicama Implements PlantInterface
{
public function sum()
{
...
}
}
Now our plant classes are cleaned up. This also gives us the ability to check if a class is implementing VitaminKInterface
, so it knows which plants have that vitamin, and the subsequent getVitaminK()
method.